description here
Deze cursus is enkel beschikbaar in het Engels. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.
This course is in French only. If this is not a problem for you, by all means go ahead and apply.

Data scientists often favor pandas, because it lets them work efficiently with larger amounts of data—a useful quality as data sets become bigger and bigger. In this course, instructor Miki Tebeka shows you how to improve your pandas’ code’s speed and efficiency. First, Miki explains why performance matters and how you can measure it with Python profilers. Then, the course teaches you how to use vectorization to manipulate data. The course also walks through some common mistakes and how to address them. Python and pandas have many high-performance built-in functions, and Miki covers how to use them. Pandas can use a lot of memory, so Miki offers good tips on how to save memory. The course demonstrates how to serialize data with SQL and HDF5. Then Miki goes over how to speed up your code with Numba and Cython. Alternative DataFrames can also speed up your code, and Miki steps through some options. Plus, explore a few extra resources that you can check out.
Topics include:
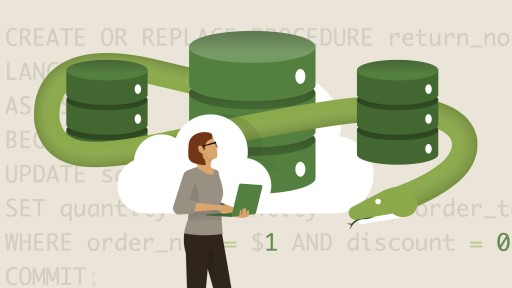
To create functional and useful Python applications, you need a database. Databases allow you to store data from user sessions, track inventory, make recommendations, and more. However, Python is compatible with many options: SQLite, MySQL, and PostgreSQL, among others. Selecting the right database is a skill that advanced developers are expected to master. This course provides an excellent primer, comparing the different types of databases that can be connected through the Python Database API. Instructor Kathryn Hodge teaches the differences between SQLite, MySQL, and PostgreSQL and shows how to use the ORM tool SQLAlchemy to query a database. The final chapters put your knowledge to practical use in two hands-on projects: developing a full-stack application with Python, PostgreSQL, and Flask and creating a data analysis app with pandas and Jupyter Notebook. By the end, you should feel comfortable creating and using databases and be able to decide which Python database is right for you.
Topics include:
- What is a database?
- Relational vs. nonrelational databases
- Creating a SQLite database
- Editing records in SQLite
- Creating a MySQL database
- Encapsulating database operations
- Creating a PostgreSQL database
- Interacting with databases using SQLAlchemy
- Creating a stored procedure
- Developing full-stack apps with Python and Flask
- Developing analysis apps with pandas and SQLAlchemy
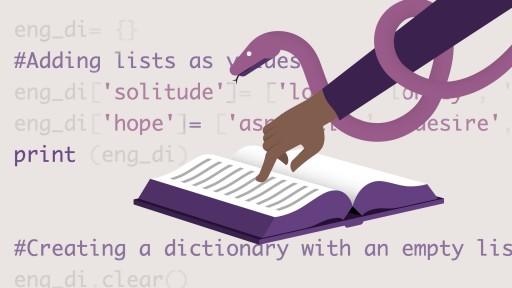
Dictionaries are a common data structure in Python programming, designed to hold a sequence of key-value pairs. Dictionaries are dynamic, can be nested, and are preferable to lists in situations where you would want to search for and retrieve data with the same key. Being able to use dictionaries effectively is critical to mastering Python and create more efficient code. In this course, Deepa Muralidhar reviews the syntax and real-world use cases for dictionaries. Discover how to create a simple dictionary, iterate through the data, incorporate operations and comparators, and compare dictionaries to other common data structures such as lists, sets, and tuples. Plus, find out how to use dictionary comprehension to automate dictionary generation by merging lists or arrays.
Topics include:
- Creating a dictionary
- Iterating through a dictionary
- Using operations and comparators
- Working with lists
- Comparing dictionaries to other data structures
- Using dictionary comprehension
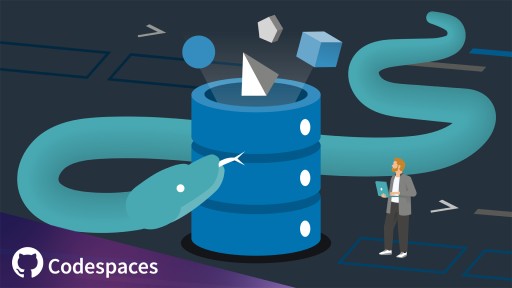
Data science is one of the fastest-growing areas of technology today. And whether you work with large data sets or just need to process spread sheet files, Python is a great language to use when working with data-intensive applications. In this course, Joe Marini gets you started on working with data in Python, highlighting some of the most useful built-in features of the language. Using a real data set from the United States Geological Survey that tracks earthquake information, Joe shows you how to perform data operations like sorting and filtering, determining basic information like minimum and maximum values, and reading and writing data to and from other formats like CSV and JSON. Check out this course with Joe to see how Python can help you make sense of your data. This course is integrated with GitHub Codespaces, an instant cloud developer environment that offers all the functionality of your favorite IDE without the need for any local machine setup. With GitHub Codespaces, you can get hands-on practice from any machine, at any time—all while using a tool that you’ll likely encounter in the workplace.
Topics include:
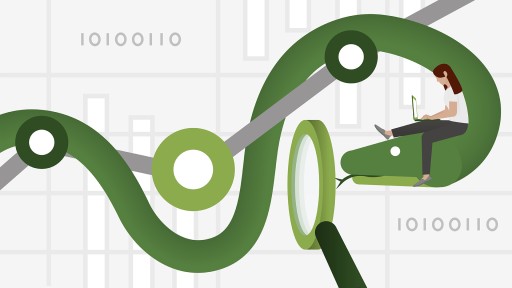
If you've worked in Python, you're likely familiar with the basic of pandas. In this advanced course, instructor Brett Vanderblock shares how you can take advantage of the advanced functions of pandas—such as working with dates, dealing with missing data, merging DataFrames, and more—to work more effectively with your data. First, Brett introduces you to DataFrames, identifies the top functions in pandas, and shows you how to configure your pandas workspace efficiently. He walks you through converting data types, working with strings, and using the apply map and applymap functions effectively. He shows you how to combine Groupby and multiple aggregate functions in pandas and how to use the merge, join, and concat functions. Brett steps through plotting and statistical functions with pandas. He concludes by explaining how you can use pandas-profiling and Geopandas to get the most out of your functions and data. This course was created by Madecraft. We are pleased to host this training in our library.
Topics include:
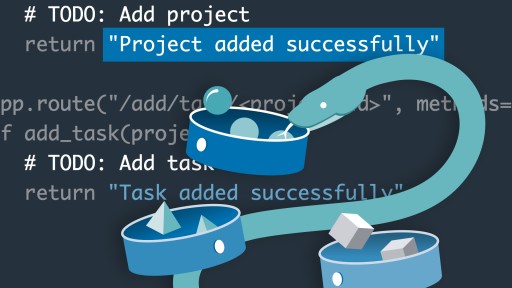
Looking for a hands-on opportunity to take your Python skills to the next level? In this course, instructor Kathryn Hodge takes you through a series of practical database examples to help level up your Python applications. Learn how to create an API that serves data from a database using FastAPI, Flask, MySQL, Postman, SQLAlchemy, endpoints, and more. Get proven tips on how to develop analysis applications with pandas, the high-performance Python library featuring robust and integrated built-in data structures. Test out your new coding skills as you go in the exercise challenges at the end of each section. By the end of this course, you’ll be ready to start building full-stack task list applications with Flask, a microframework designed uniquely for Python that lets you integrate data from a database directly to an app.
Topics include:

This is Part 1 of a series of courses intended to dive into the inner mechanics and more complicated aspects of Python 3.
This is not a beginner course!
If you've been coding Python for a week or a couple of months, you probably should keep writing Python for a bit more before tackling this series.
On the other hand, if you've been studying or programming in Python for a bit, and are now starting to ask yourself questions such as:
- I wonder how this works?
- is there another, more pythonic, way, of doing this?
- what's a closure? is that the same as a lambda?
- I know how to use a decorator someone else wrote, but how does it work? How do I write my own?
- why do some boolean expressions not return a boolean value? How can I use that to my advantage?
- how does the import mechanism in Python work, and why am I getting side effects?
- and similar types of question...
then this course is for you.
What you'll learn
- An in-depth look at variables, memory, namespaces and scopes
- A deep dive into Python's memory management and optimizations
- In-depth understanding and advanced usage of Python's numerical data types (Booleans, Integers, Floats, Decimals, Fractions, Complex Numbers)
- Advanced Boolean expressions and operators
- Advanced usage of callables including functions, lambdas and closures
- Functional programming techniques such as map, reduce, filter, and partials
- Create advanced decorators, including parametrized decorators, class decorators, and decorator classes
- Advanced decorator applications such as memoization and single dispatch generic functions
- Use and understand Python's complex Module and Package system
- Idiomatic Python and best practices
- Understand Python's compile-time and run-time and how this affects your code
- Avoid common pitfalls
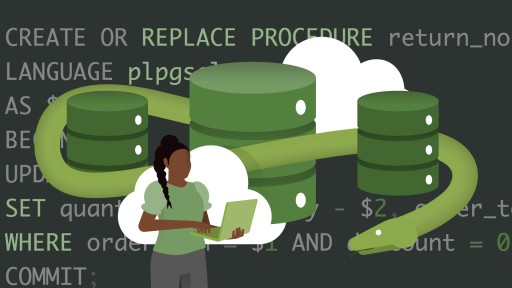
To create functional and useful Python applications, you need a database. Databases allow you to store data from user sessions, track inventory, make recommendations, and more. However, Python is compatible with many options: SQLite, MySQL, and PostgreSQL, among others. Selecting the right database is a skill that advanced developers are expected to master. This course provides an excellent primer, comparing the different types of databases that can be connected through the Python Database API. Instructor Kathryn Hodge teaches the differences between SQLite, MySQL, and PostgreSQL and shows how to use the ORM tool SQLAlchemy to query a database. The final chapters put your knowledge to practical use in two hands-on projects: developing a full-stack application with Python, PostgreSQL, and Flask and creating a data analysis app with pandas and Jupyter Notebook. By the end, you should feel comfortable creating and using databases and be able to decide which Python database is right for you.
Topics include:

Rock your next technical interview by using Python to code some of the most common linear data structures: singly and doubly linked lists. In this course, instructor Erin Allard digs into the subject of linked lists, sharing what you need to know to communicate your understanding of this data structure to an interviewer. Erin goes over abstract data types and helps you conceptualize nodes in linked lists. She also discusses how to create both the singly linked list and doubly linked list classes and goes over how to implement key methods. Throughout the course, she not only shows how to code the class and methods for each data structure, but also explains why each method is needed.
Topics include:
- Abstract data types
- Identifying the list data type and its uses
- Operations on linked lists
- Creating the singly linked list class and its methods
- Creating the doubly linked list class and its methods

Part 2 of this Python 3: Deep Dive series is an in-depth look at:
- sequences
- iterables
- iterators
- generators
- comprehensions
- context managers
- generator based coroutines
I will show you exactly how iteration works in Python - from the sequence protocol, to the iterable and iterator protocols, and how we can write our own sequence and iterable data types.
We'll go into some detail to explain sequence slicing and how slicing relates to ranges.
We look at comprehensions in detail as well and I will show you how list comprehensions are actually closures and have their own scope, and the reason why subtle bugs sometimes creep in to list comprehensions that we might not expect.
We'll take a deep dive into the itertools module and look at all the functions available there and how useful (but overlooked!) they can be.
We also look at generator functions, their relation to iterators, and their comprehension counterparts (generator expressions).
Context managers, an often overlooked construct in Python, is covered in detail too. There we will learn how to create and leverage our own context managers and understand the relationship between context managers and generator functions.
Finally, we'll look at how we can use generators to create coroutines.
Each section is followed by a project designed to put into practice what you learn throughout the course.
This course series is focused on the Python language and the standard library. There is an enormous amount of functionality and things to understand in just the standard CPython distribution, so I do not cover 3rd party libraries - this is a Python deep dive, not an exploration of the many highly useful 3rd party libraries that have grown around Python - those are often sufficiently large to warrant an entire course unto themselves! Indeed, many of them already do!
***** Prerequisites *****
Please note that this is a relatively advanced Python course, and a strong knowledge of some topics in Python is required.
In particular you should already have an in-depth understanding of the following topics:
- functions and function arguments
- packing and unpacking iterables and how that is used with function arguments (i.e. using *)
- closures
- decorators
- Boolean truth values and how any object has an associated truth value
- named tuples
- the zip, map, filter, sorted, reduce functions
- lambdas
- importing modules and packages
You should also have a basic knowledge of the following topics:
- various data types (numeric, string, lists, tuples, dictionaries, sets, etc)
- for loops, while loops, break, continue, the else clause
- if statements
- try...except...else...finally...
- basic knowledge of how to create and use classes (methods, properties) - no need for advanced topics such as inheritance or meta classes
- understand how certain special methods are used in classes (such as __init__, __eq__, __lt__, etc)
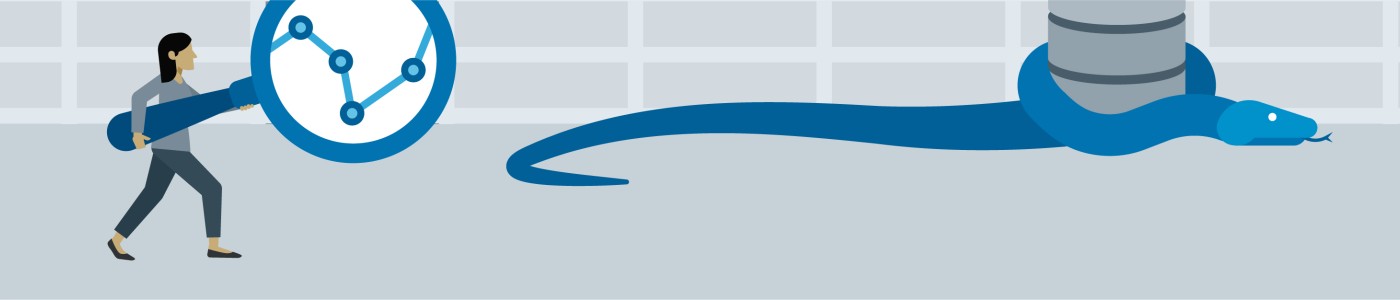
Quickly learn the general programming principles and methods for Python, and then begin applying that knowledge to using Python in data science-related development.
Topics include:
- Learn the basics of Python as an object-oriented programming language.
- Apply Python coding skills to analytics uses.
- Explore the Python scientific stack of tools.
Deze cursus is enkel beschikbaar in het Engels. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.
This course is in French only. If this is not a problem for you, by all means go ahead and apply.

This course is an in-depth look at Python dictionaries.
Dictionaries are ubiquitous in Python. Classes are essentially dictionaries, modules are dictionaries, namespaces are dictionaries, sets are dictionaries and many more.
In this course we'll take an in-depth look at:
- associative arrays and how they can be implemented using hash maps
- hash functions and how we can leverage them for our own custom classes
- Python dictionaries and sets and the various operations we can perform with them
- specialized dictionary structures such as OrderedDict and how it relates to the built-in Python3.6+ dict
- Python's implementation of multi-sets, the Counter class
- the ChainMap class
- how to create custom dictionaries by inheriting from the UserDict class
- how to serialize and deserialize dictionaries to JSON
- the use of schemas in custom JSON deserialization
- a brief introduction to some useful libraries such as JSONSchema, PyYaml and Serpy

This Python3: Deep Dive Part 4 course takes a closer look at object oriented programming (OOP) in Python.
MAIN COURSE TOPICS
- what are classes and instances
- class data and function attributes
- properties
- instance, class and static methods
- polymorphism and the role special functions play in this
- single inheritance
- slots
- the descriptor protocol and its relationship to properties and functions
- enumerations
- exceptions
- metaprogramming (including metaclasses)
What you'll learn
- Python Object Oriented Concepts
- Classes
- Methods and Binding
- Instance, Class and Static Methods
- Properties
- Property Decorators
- Single Inheritance
- Slots
- Descriptors
- Enumerations
- Exceptions
- Metaprogramming
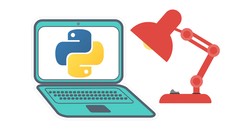
Learn Python like a Professional Start from the basics and go all the way to creating your own applications and games. This course will teach you Python in a practical manner, with every lecture comes a full coding screencast and a corresponding code notebook! In this course, you will learn:
- You will learn how to leverage the power of Python to solve tasks.
- You will build games and programs that use Python libraries.
- You will be able to use Python for your own work problems or personal projects.
- You will create a portfolio of Python based projects you can share.
- Learn to use Python professionally, learning both Python 2 and Python 3!
- Create games with Python, like Tic Tac Toe and Blackjack!
- Learn advanced Python features, like the collections module and how to work with timestamps!
- Learn to use Object Oriented Programming with classes!
- Understand complex topics, like decorators.
- Understand how to use both the Jupyter Notebook and create .py files
- Get an understanding of how to create GUIs in the Jupyter Notebook system!
- Build a complete understanding of Python from the ground up!

A practical programming course for office workers, academics, and administrators who want to improve their productivity. Automate the Boring Stuff with Python was written for people who want to get up to speed writing small programs that do practical tasks as soon as possible. In this course, you will learn:
- Automate tasks on their computer by writing simple Python programs.
- Write programs that can do text pattern recognition with "regular expressions".
- Programmatically generate and update Excel spreadsheets.
- Parse PDFs and Word documents.
- Crawl web sites and pull information from online sources.
- Write programs that send out email notifications.
- Use Python's debugging tools to quickly figure out bugs in your code.
- Programmatically control the mouse and keyboard to click and type for you.
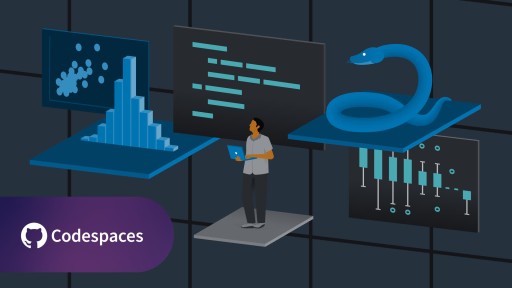
The field of statistics has become increasingly dependent on data analysis and interpretation using Python. With the rise of big data and data science, the demand for professionals who can effectively analyze and interpret data using Python has skyrocketed. In this course, Matt Harrison teaches you how to collect, clean, analyze, and visualize data using the powerful tools of the Python programming language. Join Matt as he gives into the various techniques that form the backbone of statistics and helps you understand the data with summary statistics and visualizations. He explains how to create predictive models using both linear regression andXGBoost, and wraps up the course with a look at hypothesis testing. If you’re interested in exploring statistics using a code-first approach, join Matt in this course as he shows you how to use Python to unlock the power of data.
Topics include:
Deze cursus is enkel beschikbaar in het Engels. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.
This course is in French only. If this is not a problem for you, by all means go ahead and apply.

Python—the popular and highly-readable object-oriented language—is both powerful and relatively easy to learn. Whether you're new to programming or an experienced developer, this course can help you get started with Python. Joe Marini provides an overview of the installation process, basic Python syntax, and an example of how to construct and run a simple Python program. Learn to work with dates and times, read and write files, and retrieve and parse HTML, JSON, and XML data from the web.
Topics include:
Deze cursus is enkel beschikbaar in het Engels. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.
This course is in French only. If this is not a problem for you, by all means go ahead and apply.
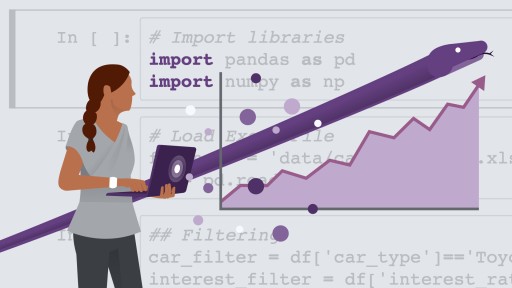
Data visualization is incredibly important for data scientists, as it helps them communicate their insights to nontechnical peers. But you don’t need to be a design pro. Python is a popular, easy-to-use programming language that offers a number of libraries specifically built for data visualization. In this course from the experts at Madecraft, you can learn how to build accurate, engaging, and easy-to-generate charts and graphs using Python. Explore the pandas and Matplotlib libraries, and then discover how to load and clean data sets and create simple and advanced plots, including heatmaps, histograms, and subplots. Instructor Michael Galarnyk provides all the instruction you need to create professional data visualizations through programming. This course was created by Madecraft. We are pleased to host this content in our library.
Topics include:
Deze cursus is enkel beschikbaar in het Engels. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.
This course is in French only. If this is not a problem for you, by all means go ahead and apply.

A sizable portion of a data scientist's day is often spent fetching and cleaning the data they need to train their algorithms. In this course, learn how to use Python tools and techniques to get the relevant, high-quality data you need. Instructor Miki Tebeka covers reading files, including how to work with CSV, XML, and JSON files. He also discusses calling APIs, web scraping (and why it should be a last resort), and validating and cleaning data. Plus, discover how to establish and monitor key performance indicators (KPIs) that help you monitor your data pipeline.
Topics include:
- Describe the characteristics of different data types and the work of data scientists.
- Describe different data serialization formats and explain how to use them in Python.
- Define APIs and explain how to use them with Python to make http calls, interpret JSON, and utilize message queues.
- Explain what web scraping is and describe ways to do it.
- Define what a schema is and describe characteristics of schemas and how they influence operations.
- Describe the characteristics of different types of databases.
- Categorize types of errors and explain how to correct them.
- Explain design criteria for data systems and describe how to monitor performance using KPIs.
Deze cursus is enkel beschikbaar in het Engels. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.
This course is in French only. If this is not a problem for you, by all means go ahead and apply.