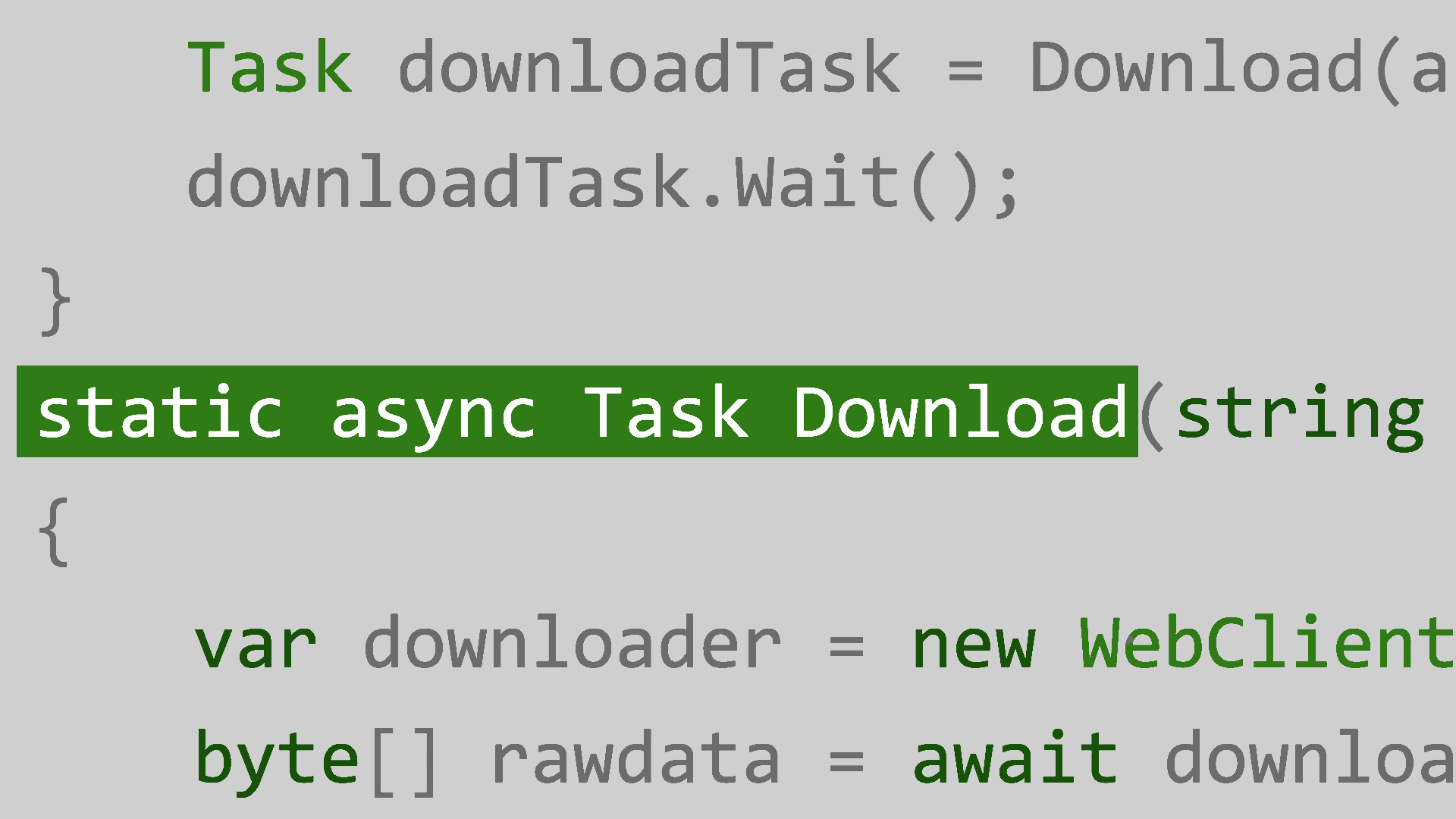
Discover how to improve the scalability and performance of your applications using asynchronous programming in C#. In this course, join Anton Delsink as he explores different options for parallelizing your code, and shows how asynchronous programming is done with C#. Anton covers key concepts, including unit tests, delegates, lambdas, and anonymous methods. He also covers multithreading, async keywords, tasks, and more.
Topics include:
- What is asynchronous?
- Delegates
- Blocking vs. nonblocking I/O
- Async database queries with begin/end
- Windows Forms BackgroundWorker
- Threads
- Async networking with Tasks
- Async database queries with Tasks
- C# keywords async and await
Deze cursus is enkel beschikbaar in het Engels. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.
Deze cursus is enkel beschikbaar in het Frans. Als dit voor u geen probleem vormt, dien dan gerust uw aanvraag in.